Create Your Custom Theme from Scratch
We provide official themes that you can customize to fit your needs. Consider customizing our themes first if you only need to make slight style changes.
However, some users may prefer to create their own custom theme entirely from scratch. You can achieve this by following these procedures:
Create Your Theme’s Default Styles: Define the fundamental styles that will form the basis of your theme.
Optional: Create Named Theme Styles: Optionally, define named theme styles such as “blue”, “blue_solid” to customize specific elements further.
Optional: Define Background Color and Source Code Font: Set preferences for background color and source code font if desired.
Apply Your Custom Theme: Pass your defined styles and preferences to
dtheme.apply_custom_theme()
to apply your custom theme.
The dtheme.apply_custom_theme()
function accepts the following arguments:
default_style
: Default styles for your theme.named_styles
(optional): Named styles within your theme, e.g., “blue”.theme_colors
(optional): List of colors used in your theme.backgroundcolor
(optional): Background color of your theme.sourcecodefont
(optional): Default font for displaying source code.
Let’s go through these steps in detail.
Create ThemeStyles
Object
The default_style
and named_styles
arguments require a dtheme.ThemeStyles
object.
This class serves as a container for Drawlib’s styles such as LineStyle
, ShapeStyle
, etc.
When defining your data, populate the default_style
object with the following mandatory items:
iconstyle
imagestyle
linestyle
linearrowstyle
shapestyle
shapetextstyle
textstyle
Other items are optional for the default_style
, and all items are optional for named_styles
.
Apply Theme Styles
Once you have defined your ThemeStyles
objects, pass them to dtheme.apply_custom_theme()
.
This function clears old theme styles internally before applying your new custom theme.
Here’s an example script demonstrating the creation of a gold and silver themed custom theme:
1from drawlib.apis import *
2
3default = dtheme.ThemeStyles(
4 iconstyle=IconStyle(style="fill", color=Colors140.Gold),
5 imagestyle=ImageStyle(lcolor=Colors140.Gold, lwidth=0),
6 linestyle=LineStyle(width=3, color=Colors140.Gold),
7 shapestyle=ShapeStyle(lwidth=0, lcolor=Colors140.Black, fcolor=Colors140.Gold),
8 shapetextstyle=ShapeTextStyle(color=Colors140.DarkRed, font=FontSansSerif.RALEWAYS_BOLD),
9 textstyle=TextStyle(color=Colors140.DarkRed, font=FontSansSerif.RALEWAYS_BOLD),
10)
11
12gold = dtheme.ThemeStyles(
13 iconstyle=IconStyle(style="fill", color=Colors140.Gold),
14 imagestyle=ImageStyle(lcolor=Colors140.Gold, lwidth=0),
15 linestyle=LineStyle(width=3, color=Colors140.Gold),
16 shapestyle=ShapeStyle(lwidth=0, lcolor=Colors140.Black, fcolor=Colors140.Gold),
17 shapetextstyle=ShapeTextStyle(color=Colors140.Gold, font=FontSansSerif.RALEWAYS_BOLD),
18 textstyle=TextStyle(color=Colors140.Gold, font=FontSansSerif.RALEWAYS_BOLD),
19)
20
21silver = dtheme.ThemeStyles(
22 iconstyle=IconStyle(style="fill", color=Colors140.Silver),
23 imagestyle=ImageStyle(lcolor=Colors140.Silver, lwidth=0),
24 linestyle=LineStyle(width=3, color=Colors140.Silver),
25 shapestyle=ShapeStyle(lwidth=0, lcolor=Colors140.Black, fcolor=Colors140.Silver),
26 shapetextstyle=ShapeTextStyle(color=Colors140.Silver, font=FontSansSerif.RALEWAYS_BOLD),
27 textstyle=TextStyle(color=Colors140.Silver, font=FontSansSerif.RALEWAYS_BOLD),
28)
29
30dtheme.apply_custom_theme(
31 default_style=default,
32 named_styles=[
33 ("gold", gold),
34 ("silver", silver),
35 ],
36 theme_colors=[
37 ("gold", Colors140.Gold),
38 ("silver", Colors140.Silver),
39 ],
40 backgroundcolor=Colors140.AliceBlue,
41 sourcecodefont=FontSourceCode.ROBOTO_MONO,
42)
43
44config(width=100, height=50)
45x1 = 20
46x2 = 50
47x3 = 80
48y1 = 10
49y2 = 25
50y3 = 40
51
52# apply default style
53line((x1 - 10, y1), (x1 + 10, y1))
54circle((x1, y2), radius=10)
55text((x1, y3), "Hello Drawlib!")
56
57# apply named style
58for x, name in [(x2, "gold"), (x3, "silver")]:
59 line((x - 10, y1), (x + 10, y1), style=name)
60 circle((x, y2), radius=10, style=name)
61 text((x, y3), "Hello Drawlib!", style=name)
62
63save()
In this example, we define ThemeStyles
for gold and silver themes and apply them using dtheme.apply_custom_theme()
.
Executing this script will produce the following output:
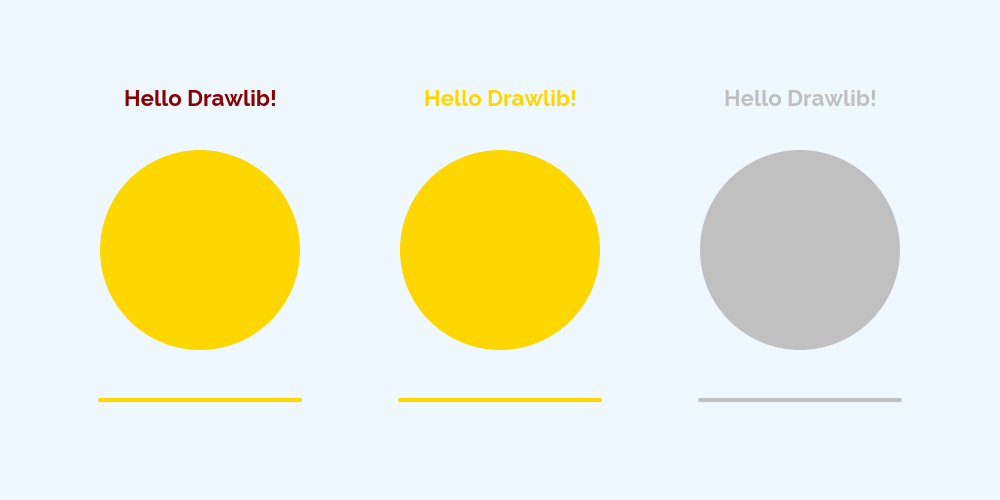
Drawing items with custom theme
You can observe that the default style of the theme has been set to gold, and the named styles “gold” and “silver” are also applied.
Recommendation
While you have the flexibility to create your own theme, it is recommended to adhere to Drawlib’s official style guidelines when sharing themes to ensure consistency and compatibility.