Drawing Text
Drawing text requires understanding the following concepts:
text(): Function for drawing text
TextStyle: Style class for text
font: How to specify fonts
Theme’s pre-defined styles
We will explain each of these concepts in this section.
text()
Drawlib uses the text()
function for drawing text.
It takes the following arguments:
xy: coordinate of drawing point
text: text value
size(optional): size of text font
angle(optional): angle of text
style(optional): text style
Font size can be changed with size
, but it can also be changed by the style
attribute.
We recommend using the style attribute rather than size.
Similar to how you shouldn’t configure text size in HTML but should do it in CSS, it’s best to handle text styling via the style attribute for better consistency and flexibility.
We will explain style later, so for now, we will focus on the other arguments. Here are three examples:
1from drawlib.apis import *
2
3config(width=100, height=50)
4text(xy=(25, 15), text="Hello Drawlib.")
5text(xy=(25, 35), text="Hello Drawlib.", size=24)
6text(xy=(75, 25), text="こんにちは Drawlib.", angle=45)
7save()
Below is a figure illustrating these examples:
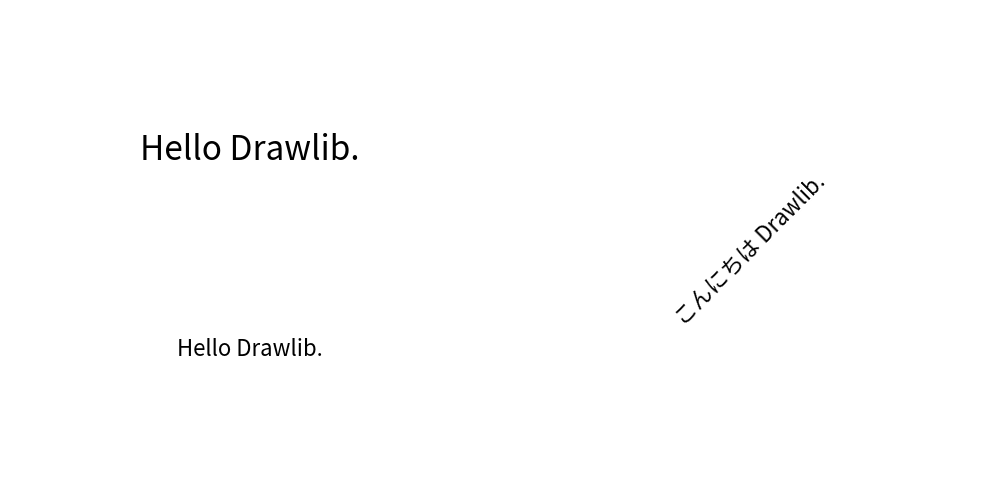
text()
Drawlib’s default theme uses the multilingual font “Noto Sans CJK Japanese”. This is a popular sans-serif font that supports CJK (Chinese, Japanese, Korean) in addition to the alphabet. As the font name suggests, it prioritizes Japanese but typically does not conflict with Chinese and Korean.
Fonts that prioritize Chinese and Korean are also provided. Additionally, other local language fonts are available for languages not supported by the default font.
text_vertical()
While it is not common in alphabetic languages, vertically aligned text is popular in a few languages, such as Japanese.
We provide the text_vertical()
function to achieve this.
The arguments are exactly the same as those for text()
.
xy: coordinate of drawing point
text: text value
size(optional): size of text font
angle(optional): angle of text
style(optional): text style
Here are three examples:
1from drawlib.apis import *
2
3config(width=100, height=50)
4text_vertical(xy=(15, 25), text="Hello Drawlib.")
5text_vertical(xy=(35, 25), text="Hello Drawlib.", size=12)
6text_vertical(xy=(75, 25), text="こんにちは Drawlib.", angle=45)
7save()
Below is a figure illustrating these examples:
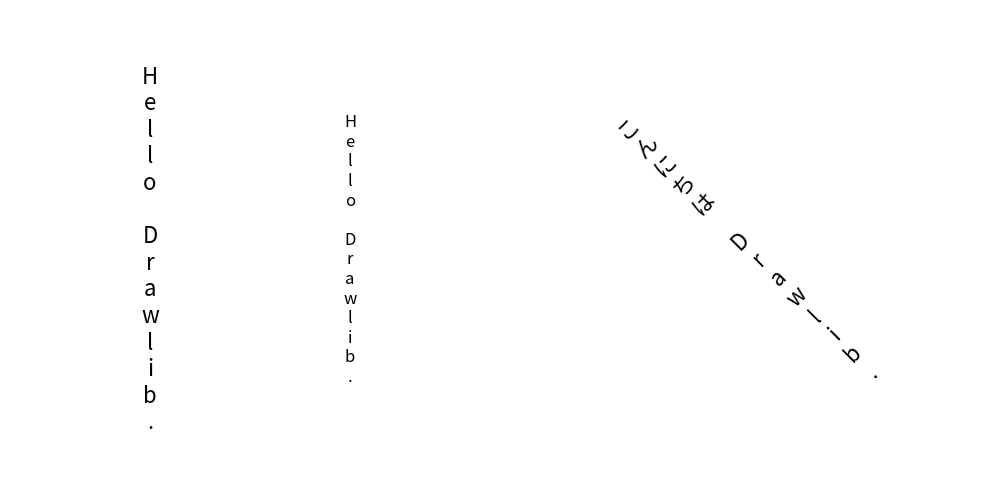
text_vertical()
Please use halign="center"
in TextStyle.
It is the default value.
Horizontal align left/right will work, but it does not look nice except with monospaced fonts.
TextStyle
TextStyle
is style class for text()
and text_vertical()
.
It encompasses many attributes, categorized into “alignment”, “text style”, and “text background style”.
TextStyle
has these attributes.
halign: Horizontal alignment of text. Options are “left”, “center”, “right”.
valign: Vertical alignment of text. Options are “bottom”, “center”, “top”.
color: Text color.
size: Text size.
font: Text font.
bgalpha: Background alpha.
bglwidth: Background line width.
bglcolor: Background line color.
bglstyle: Background line style. Options are “solid”, “dashed”, “dotted”, “dashdot”.
bgfcolor: Background fill color.
The default alignment is “center” horizontally and “center” vertically. By default, no background is drawn.
Here are 2 examples.
1from drawlib.apis import *
2
3config(width=100, height=50, grid_only=True)
4text(
5 xy=(15, 25),
6 text="Hello Drawlib.",
7 style=TextStyle(
8 color=Colors140.Turquoise,
9 size=24,
10 halign="left",
11 valign="bottom",
12 font=FontSerif.MERRIWEATHER_REGULAR,
13 ),
14)
15circle(xy=(15, 25), radius=0.5, style=ShapeStyle(fcolor=Colors.Red, lwidth=0))
16text((15, 22), "align: left,bottom")
17
18text(
19 xy=(75, 25),
20 angle=45,
21 text="こんにちは Drawlib.",
22 style=TextStyle(
23 color=Colors.White,
24 bglwidth=2,
25 bglcolor=Colors.Red,
26 bglstyle="dashed",
27 bgfcolor=Colors.Black,
28 ),
29)
30save()
The left-side example configures alignment and text style, while the right-side example configures text background style. Below is a figure illustrating these examples:
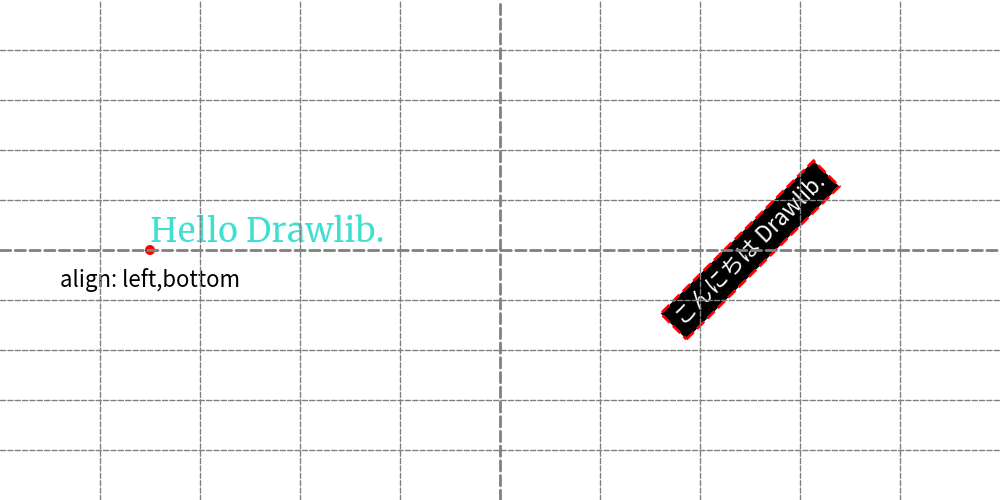
TextStyle
In our opinion, there are few chances to use text background. Setting a white (or another canvas background color) background without a border can be useful for drawing text over shapes and lines in some situations.
Font
Drawlib specifies fonts from Drawlib’s font library or from your own font files. Drawlib does not use system fonts installed on your PC because using system fonts may result in inconsistent rendering across different environments.
Basic Font Classes
Drawlib includes these basic Font classes:
Font
FontSanSerif
FontSerif
FontMonoSpace
FontSourceCode
FontRoboto
These classes contain popular fonts or fonts preferred by the Drawlib team.
Local Language Fonts
Additionally, Drawlib supports local language fonts:
FontArabic
FontBrahmic
FontChinese
FontJapanese
FontKorean
FontThai
If your preferred language is not supported, please let us know. However, we can only support open-source free fonts at the moment. And currently, we use Google fonts.
Here are font examples.
1from drawlib.apis import *
2
3config(width=100, height=60, grid_only=True)
4text(xy=(25, 5), text="Hello Drawlib.", style=TextStyle(font=Font.SANSSERIF_LIGHT))
5text(xy=(25, 15), text="Hello Drawlib.", style=TextStyle(font=Font.SANSSERIF_REGULAR))
6text(xy=(25, 25), text="Hello Drawlib.", style=TextStyle(font=Font.SANSSERIF_BOLD))
7text(xy=(25, 35), text="Hello Drawlib.", style=TextStyle(font=Font.SERIF_LIGHT))
8text(xy=(25, 45), text="Hello Drawlib.", style=TextStyle(font=Font.SERIF_REGULAR))
9text(xy=(25, 55), text="Hello Drawlib.", style=TextStyle(font=Font.SERIF_BOLD))
10
11text(xy=(75, 5), text="Hello Drawlib.", style=TextStyle(font=FontRoboto.ROBOTO_REGULAR))
12text(xy=(75, 15), text="Hello Drawlib.", style=TextStyle(font=FontSansSerif.RALEWAYS_REGULAR))
13text(xy=(75, 25), text="Hello Drawlib.", style=TextStyle(font=FontSerif.MERRIWEATHER_REGULAR))
14text(xy=(75, 35), text="Hello Drawlib.", style=TextStyle(font=FontMonoSpace.SOURCECODEPRO_REGULAR))
15text(xy=(75, 45), text="こんにちは Drawlib.", style=TextStyle(font=FontJapanese.MPLUS1P_REGULAR))
16text(xy=(75, 55), text="สวัสดี ดรอว์ลิบ", style=TextStyle(font=FontThai.SERIF_REGULAR))
17
18save()
Below is a figure illustrating these examples:
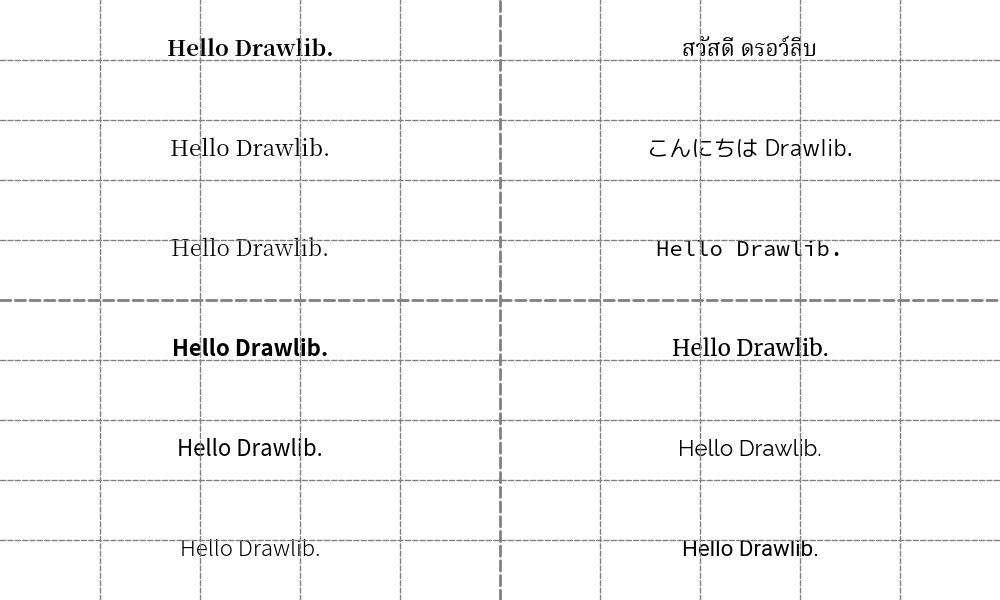
Fonts
To change the default font, refer to the theme documentation.
The dtheme.allstyles.merge()
function allows you to partially override pre-defined styles.
If you provide a TextStyle with fonts, the pre-defined fonts will be overridden with your specified font.
Custom Font
Drawlib offers a wide range of fonts.
However, if you want to use fonts that are not supported, use the FontFile
class.
This class can be used in place of the basic font classes and accepts a font file as an argument.
Here is an examples which uses font avenger.
1from drawlib.apis import *
2
3config(width=100, height=50)
4text(
5 (50, 25),
6 "Hello Drawlib!",
7 style=TextStyle(
8 size=36,
9 font=FontFile("./avenger/regular.ttf"),
10 ),
11)
12save()
Below is a figure illustrating these examples:
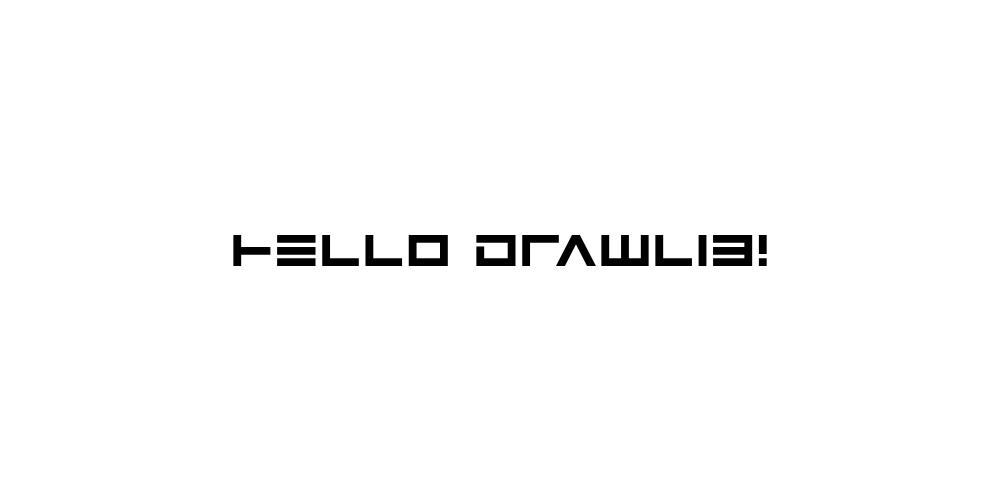
FontFile
You can check the list of fonts supported by Drawlib in the Font documentation.
Theme’s pre-defined styles
Text in Drawlib can utilize pre-defined styles from the theme you select.
The style syntax is: <color>_<type>_<weight>
.
If the color and weight are default, they are not explicitly shown in the style name.
However, text styles do not use the type variations that are used for lines and shapes.
Each weight type variation includes different font weights:
light
: Font weight lightdefault: Font weight regular
bold
: Font weight bold
Here is an example script that demonstrates the use of theme-defined text styles:
1from drawlib.apis import *
2
3config(width=100, height=50)
4text(xy=(25, 15), text="Hello Drawlib.", style="red")
5text(xy=(25, 35), text="Hello Drawlib.", size=12, style="bold")
6text(xy=(75, 15), text="Hello Drawlib.", style="blue_light")
7text(xy=(75, 35), text="Hello Drawlib.", size=24, style="green_bold")
8save()
Below is a figure illustrating these examples:
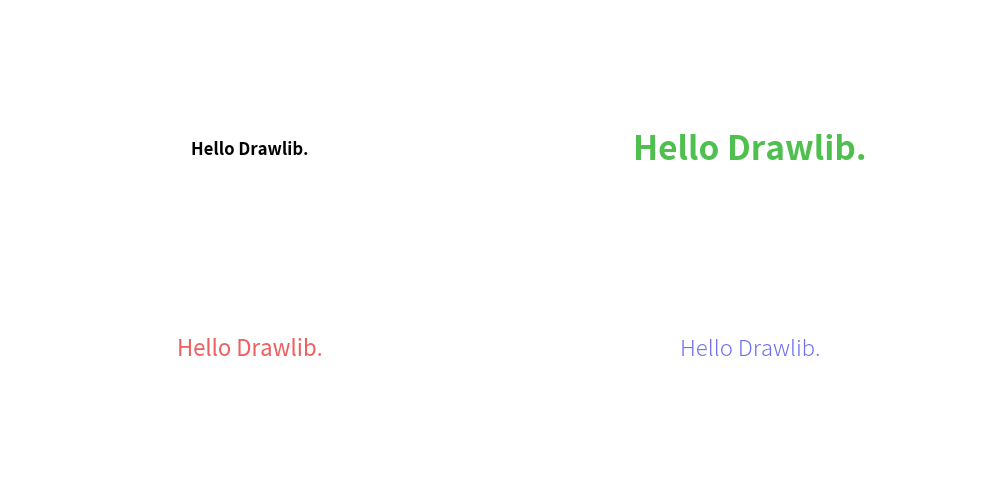
Theme’s pre-defined styles