SourceCode
Drawing source code with Drawlib can be done simply using the text()
function with halign="left"
and a monospace font.
For more sophisticated source code visuals, including syntax highlighting, Drawlib provides the SourceCode Smart Art feature.
This feature leverages pygments to generate visually enhanced source code images.
Here is an example of code:
1from drawlib.apis import *
2
3CODE = """
4from drawlib.apis import *
5
6config(width=100, height=100)
7circle(
8 xy=(50, 50),
9 radius=30,
10 style=ShapeStyle(
11 lstyle="dashed",
12 lcolor=Colors140.BlueViolet,
13 lwidth=5,
14 fcolor=Colors140.Turquoise,
15 ),
16)
17save()
18""".strip()
19
20config(width=100, height=50)
21
22sc1 = dsart.SourceCode(
23 language="python",
24 style="default",
25)
26sc1.draw((25, 25), width=40, code=CODE)
27
28sc2 = dsart.SourceCode(
29 style="monokai",
30 font=FontSourceCode.ROBOTO_MONO,
31 show_linenum=True,
32 linenum_textcolor=Colors140.Black,
33 linenum_bgcolor=Colors140.LightGray,
34)
35sc2.draw((75, 25), width=40, code=CODE, style=ImageStyle(lwidth=2, lcolor=Colors.Red))
36
37save()
In the example above, the dsart.SourceCode
instance is configured with options such as:
language: Specifies the programming language (automatically detected if not provided).
style: Defines the syntax highlighting style (e.g., monokai).
font: Source code font
show_linenum: Determines whether to display line numbers.
linenum_textcolor and linenum_bgcolor: Customize the colors of line numbers.
After creating instance, you will draw code with draw()
method.
This method’s arg is same to image()
.
But it takes code
argument instead of image
argument.
Here is a list of draw()
arguments:
xy: Coordinates to place the source code image.
width: Width of the source code image.
code: The source code string.
style: ImageStyle for source code image
Executing the code will generate below output:
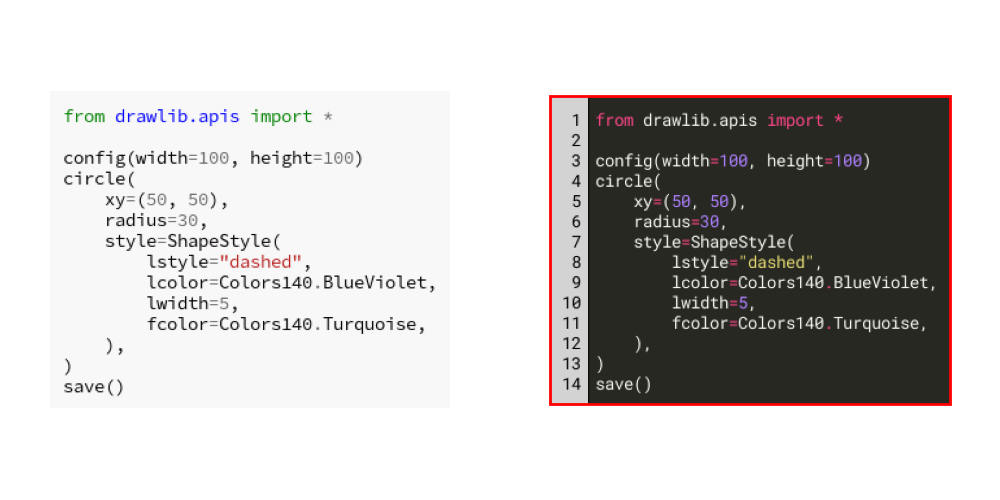
image1.png
Source Code Styles
Drawlib supports a variety of styles from Pygment’s recommended list, as well as additional black and white styles. Here are list of supported styles:
bw
sas
staroffice
xcode
default
monokai
lightbulb
github-dark
rrt
algol
algol_nu
friendly_grayscale
Here are output of Source Code styles.
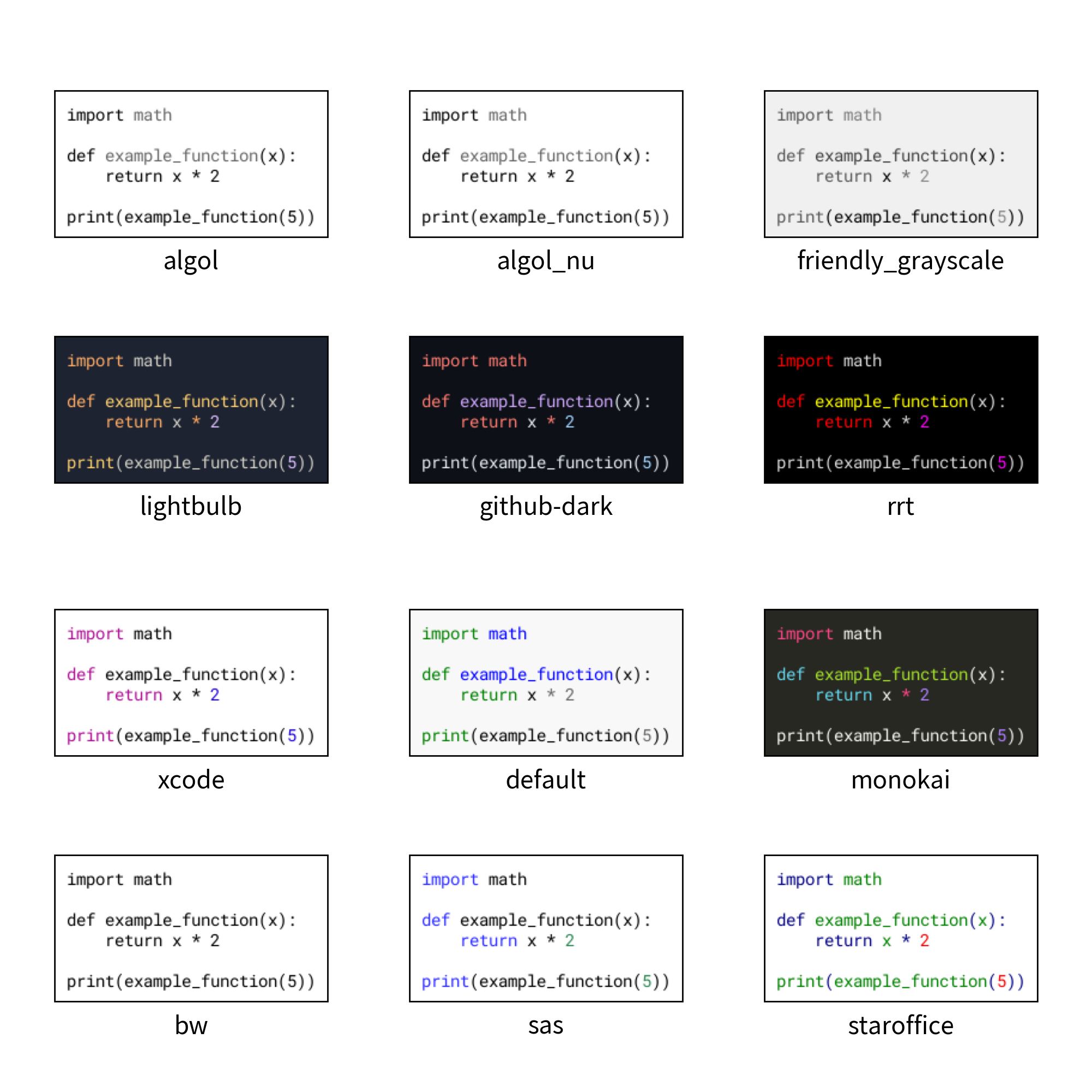
image2.png
get_text()
To retrieve the text content directly from a code file, you can use the dsart.SourceCode.get_text()
method provided by SourceCode.
This function allows you to specify a file path relative to the code file’s location.