Theme feature
Drawlib possesses a theme feature. The default theme is applied automatically at the start.
A theme is a combination of predefined styles.
If you don’t specify a style for drawing items, the predefined theme style will be applied.
Alternatively, you can specify a style with a shortcut, such as text((10, 10), "Hello", style="blue")
.
Understanding drawlib’s theme feature will save you from defining many style objects. Additionally, your illustrations will achieve consistency in styles.
Here are the key concepts of drawlib’s theme system:
The theme feature exists under dtheme.
Official themes are available. The default theme is default.
Each theme has default styles for drawing items.
Other theme styles can be used with keywords.
You can modify existing themes.
You can create your own new themes.
We will cover the basics in this document. Advanced topics are covered in the theme chapter of the documentation.
Get List of Official Themes
Drawlib possesses a few official themes.
The default theme is default
.
To use another theme, you need to specify its name.
You can get a list of official theme names with dtheme.list_official_themes()
function.
Here is an example.
1from drawlib.apis import *
2
3official_theme_names = dtheme.list_official_themes()
4print(official_theme_names)
5# ["default", "essentials", "monochrome"]
As you can see, we supports these themes now.
"default"
"essentials"
"monochrome"
Apply Official Themes
Here is a circle with the default (not specified) theme.
1from drawlib.apis import *
2
3config(width=100, height=50)
4circle((50, 25), radius=15)
5save()
Executing this code yields the following image:
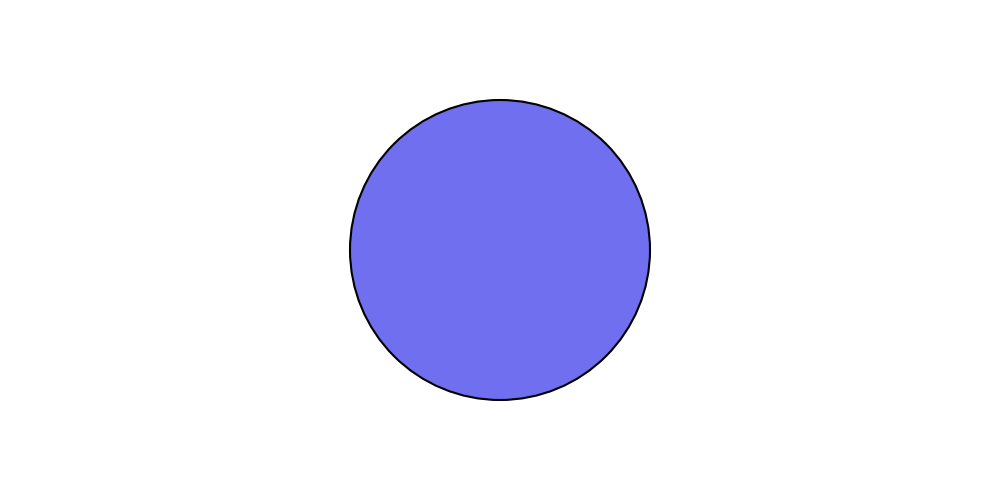
theme “default”
As you can see, the circle color is light blue.
If you don’t provide any style, the theme’s default style is applied.
This is the default style of the default
theme.
Let’s change the theme to monochrome
.
The dtheme.apply_official_theme()
function takes the theme name as an argument.
This will change the theme accordingly.
Here is an example code:
1from drawlib.apis import *
2
3dtheme.apply_official_theme("monochrome")
4config(width=100, height=50)
5circle((50, 25), radius=15)
6save()
The only difference in the code is specifying the theme. Executing this code yields the following image:
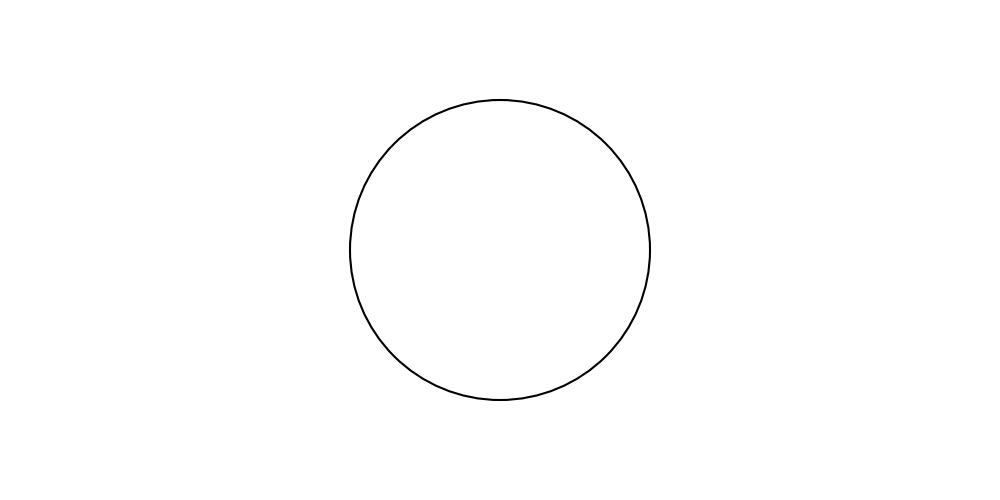
theme “monochrome”
You can see a black outlined circle.
This is the default style of the monochrome
theme.
Notice
The function clear()
will initialize the drawing state, but theme
and Dimage.cache
are exceptions.
They will be kept even if clear()
is called.
Consider this: you create your own styles and want to apply them to many illustration codes.
clear()
must be called between executing illustration codes.
Clearing styles each time you draw might cause frustration.
Therefore, it doesn’t clear styles and caches.
To clear the theme and cache, please call dutil_canvas.initialize()
.
This will initialize all canvas states.
Theme’s Pre-defined Style names
In the previous example, we examined each theme’s default styles. As mentioned, the default style is applied when no style is provided.
You can apply a theme’s style to drawing items using its name.
Drawing functions accept the style argument, which can take style classes like ShapeStyle
and TextStyle
, as well as string style names.
If the name exists, the corresponding style will be applied; otherwise, an error will occur.
The theme default
includes the following style names:
"" (blank)
: Default style applied when no style name is provided."red"
: Light blue color"green"
: Light green color"blue"
: Light blue color"black"
: Black color"white"
: White color
Let’s see them in action.
1from drawlib.apis import *
2
3config(width=100, height=50)
4line_y = 40
5text_y = 10
6
7# default style
8line((13, line_y), (27, line_y))
9circle((20, 25), radius=8)
10text((20, text_y), text="no style")
11
12# blue style
13line((33, line_y), (47, line_y), style="blue")
14circle((40, 25), radius=8, style="blue")
15text((40, text_y), text='style="blue"', style="blue")
16
17# green style
18line((53, line_y), (67, line_y), style="green")
19circle((60, 25), radius=8, style="green")
20text((60, text_y), text='style="green"', style="green")
21
22# red style
23line((73, line_y), (87, line_y), style="red")
24circle((80, 25), radius=8, style="red")
25text((80, text_y), text='style="red"', style="red")
26
27save()
In the example above, "blue"
, "green"
, and "red"
are provided as style names.
This method simplifies specifying styles by name rather than creating style objects.
Executing this code produces the following image:
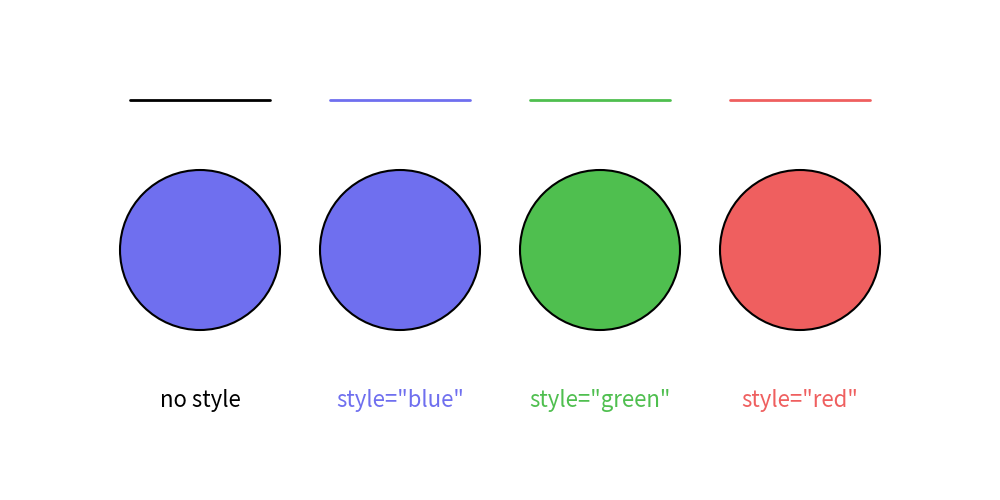
Specifying theme style name
You can view the available style names using dtheme.print_style_table()
.
1from drawlib.apis import *
2
3dtheme.print_style_table()
4# +----------------+---+-----+-------+------+-------+-------+
5# | class \ name | | red | green | blue | black | white |
6# +----------------+---+-----+-------+------+-------+-------+
7# | IconStyle | x | x | x | x | x | x |
8# | ImageStyle | x | x | x | x | x | x |
9# | LineStyle | x | x | x | x | x | x |
10# | ShapeStyle | x | x | x | x | x | x |
11# | ShapeTextStyle | x | x | x | x | x | x |
12# | TextStyle | x | x | x | x | x | x |
13# +----------------+---+-----+-------+------+-------+-------+
This will print a matrix showing style names and the supported style classes. For instance, theme default’s style names can be used across all style classes.
Style Name Rules
We uses color style names at last example, however other style names are also provided. Before delving into the rules, let’s examine them, with an example:
1from drawlib.apis import *
2
3config(width=100, height=50)
4line_y = 40
5text_y = 10
6
7# default style
8line((8, line_y), (22, line_y), style="blue")
9circle((15, 25), radius=8, style="blue")
10text((15, text_y), text='style="blue"', style="blue")
11
12# blue style
13line((31, line_y), (45, line_y), style="blue_solid")
14circle((38, 25), radius=8, style="blue_solid")
15text((38, text_y), text='style="blue_solid"')
16
17# green style
18line((55, line_y), (69, line_y), style="blue_bold")
19circle((62, 25), radius=8, style="blue_bold")
20text((62, text_y), text='style="blue_bold"', style="blue_bold")
21
22# pink style
23line((78, line_y), (92, line_y), style="dashed")
24circle((85, 25), radius=8, style="dashed")
25text((85, text_y), text='style="dashed"')
26
27save()
Executing this code results in the following image:
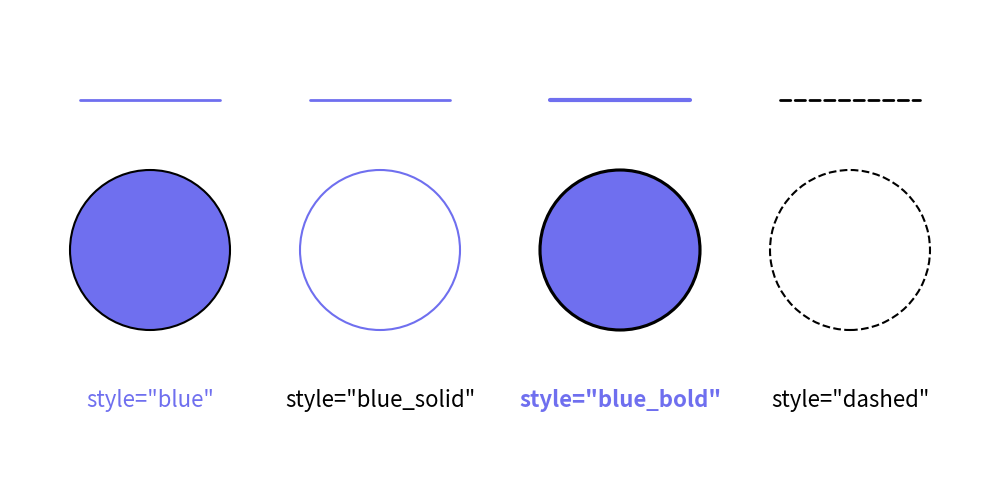
Specifying theme style name
The style names follow this syntax: <color>_<type>_<weight>
.
If the color, type, and weight are default, they may not appear in the style name.
Each style type has variations for line and fill styles with ImageStyle
, ShapeStyle
, LineStyle
.
IconStyle
supports only default and flat``(fill) style types.
``TextStyle
, ShapeTextStyle
cannot use these styles.
default: Has border and fill color
flat
: Has no bordersolid
: Shape has an outline but no filldashed
: Dashed outline, no fill
Each weight types has variation of line width for ImageStyle
, ShapeStyle
, LineStyle
.
On TextStyle
and ShapeTextStyle
, it refers to font weight:
light
: Half of the default line width; font is lightdefault: Regular line width; font is regular
bold
: Double the default line width; font is bold
Here is a matrix illustrating style types and weight:
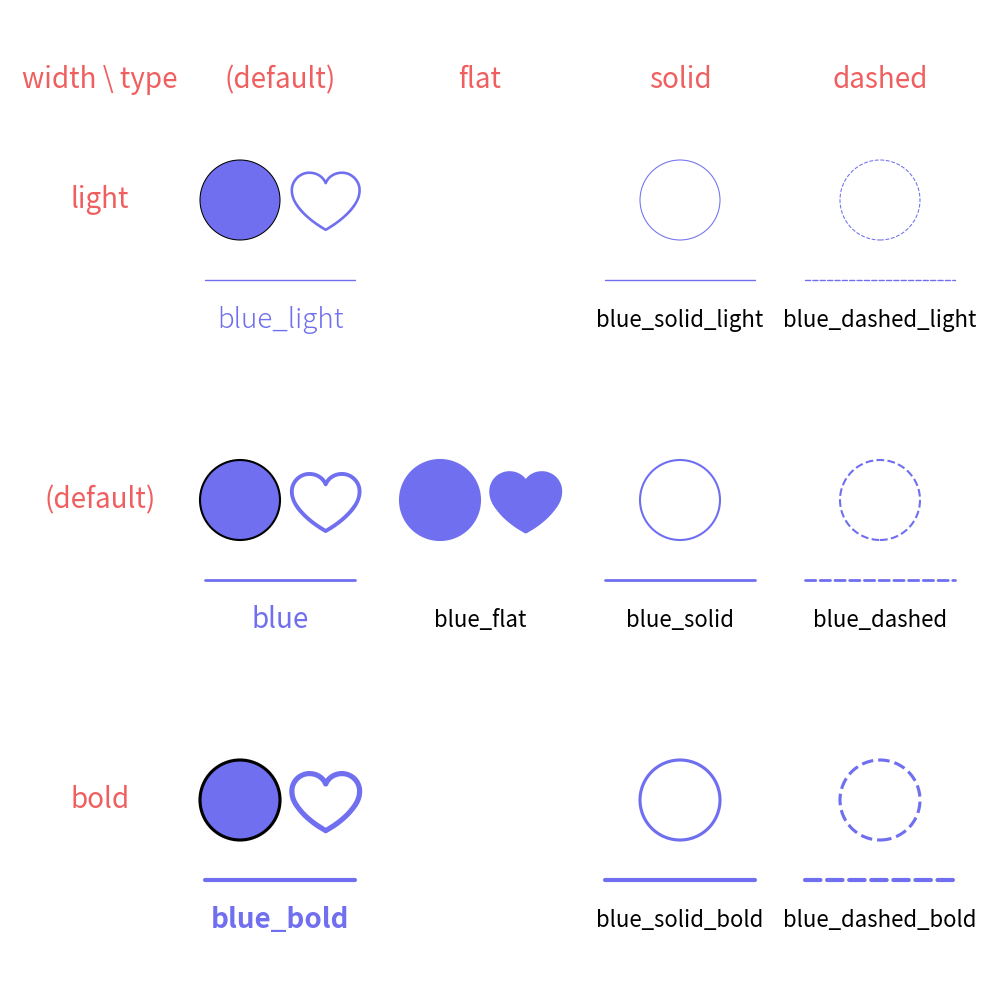
Matrix of style type and weight
In this matrix example, we use only the color "blue"
, but other colors are also available.
Note that ImageStyle
and ShapeTextStyle
are not explicitly shown, but they follow similar principles to ShapeStyle
and TextStyle
regarding theme styles.
Understanding these rules is key to mastering drawlib’s theme styles. For detailed information on each theme, please refer to the theme chapter.