Coordinate and Alignment
Drawing items accurately requires precise positioning. Understanding drawlib’s coordinates and alignment is essential to achieve this.
Coordinate
Nearly all drawing functions include arguments like xy
or similar, such as xy1
or xys
.
These represent the tuple (X, Y)
coordinate of your drawing item.
Here, X
represents the x-axis value, and Y
represents the y-axis value.
Let’s delve into some code examples:
1from drawlib.apis import *
2
3config(width=10, height=10, grid_only=True)
4for i in range(11):
5 circle(xy=(i, i), radius=0.2)
6save()
In this example, setting config(width=10, height=10, ...)
implies:
x-axis: 0 to 10
y-axis: 0 to 10
Both axes always start from 0. In this scenario, the bottom-left is (0, 0), and the top-right is (10, 10). Within the for loop, we plot small circles from coordinates (0, 0) to (10, 10).
Executing this code generates the following image:
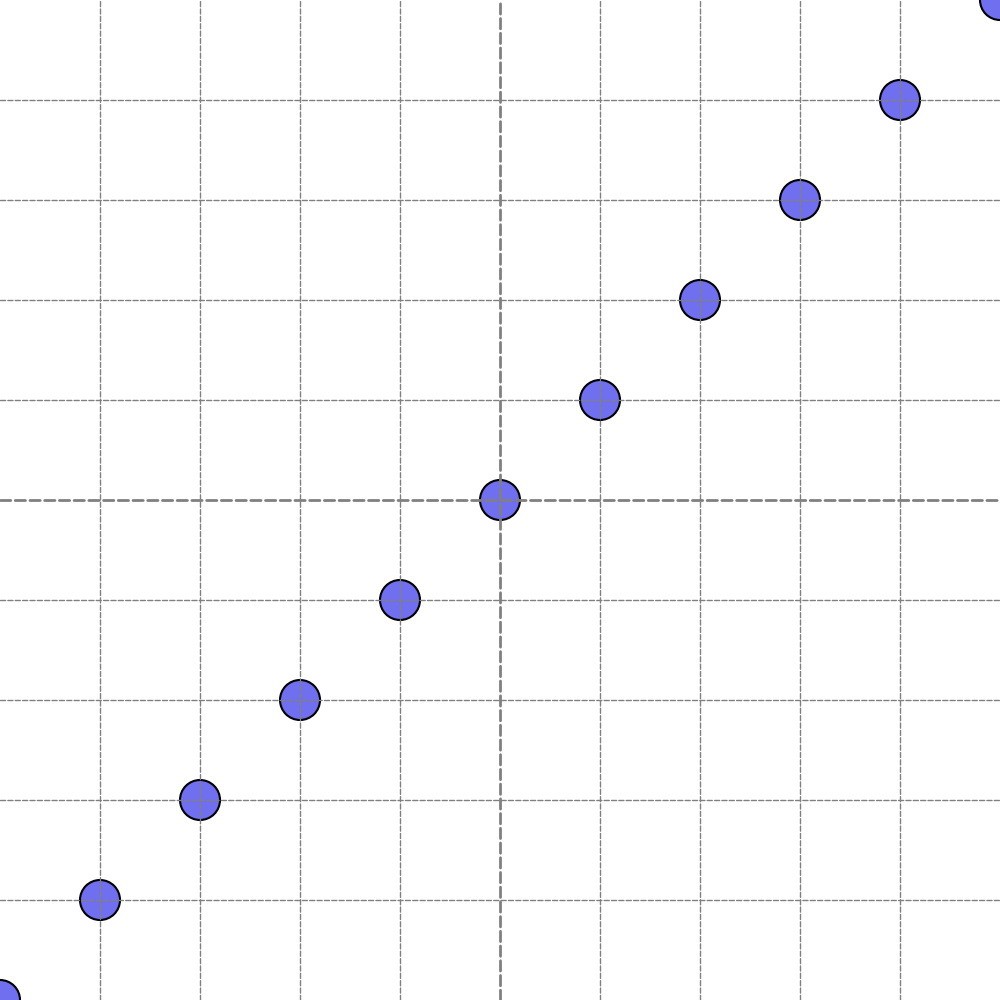
Circles from (0, 0) to (10, 10)
As you observe, (0, 0) represents the minimum value. Therefore, the circle at (0, 0) is only partially displayed. Shapes existing at values less than 0 are not drawn, and similarly, those exceeding the maximum value of 10 are omitted.
The default values for width and height are both 100
.
We recommend explicitly setting width and height using config() even if you’re using default values to showcase the image’s coordinate size.
You may need to calculate coordinates either mentally or programmatically within drawlib’s code for drawing objects.
It’s advisable to use simple values such as 100 to simplify calculations.
Setting complex values like 1920 can complicate matters.
We prefer using config(width=100, height=100)
or config(width=100, height=50)
.
Alignment
Alignment refers to the arrangement of items, such as text, images, or shapes, on drawlib’s canvas.
These items can be aligned horizontally and vertically.
The default alignment is center horizontally and center vertically.
You can alter alignment using Style
classes for each drawing item:
Icon:
IconStyle
Image:
ImageStyle
Line:
LineStyle
andLineArrowStyle
Shape:
ShapeStyle
andShapeTextStyle
Text:
TextStyle
All of these have attributes:
halign
: Horizontal alignmentvalign
: Vertical alignment
By setting values "left"
, "center"
, or "bottom"
to halign and "bottom"
, "center"
, or "top"
to valign, you can adjust the drawing item’s alignment.
If alignment isn’t specified, "center"
is applied to both halign and valign.
Let’s examine the alignment of rectangles with an example code:
1from drawlib.apis import *
2
3config(width=100, height=100, grid_only=True)
4
5for x, halign in [(15, "left"), (50, "center"), (85, "right")]:
6 for y, valign in [(15, "bottom"), (50, "center"), (85, "top")]:
7 rectangle(
8 xy=(x, y),
9 width=15,
10 height=15,
11 style=ShapeStyle(halign=halign, valign=valign),
12 text=f"({halign}, {valign})",
13 textstyle=ShapeTextStyle(size=14),
14 )
15 circle(
16 xy=(x, y),
17 radius=1,
18 style=ShapeStyle(lcolor=Colors.Red, fcolor=Colors.Red),
19 )
20
21save()
In this code, we display nine variations of alignments. The red dot represents “xy”, and the inner text indicates the alignment.
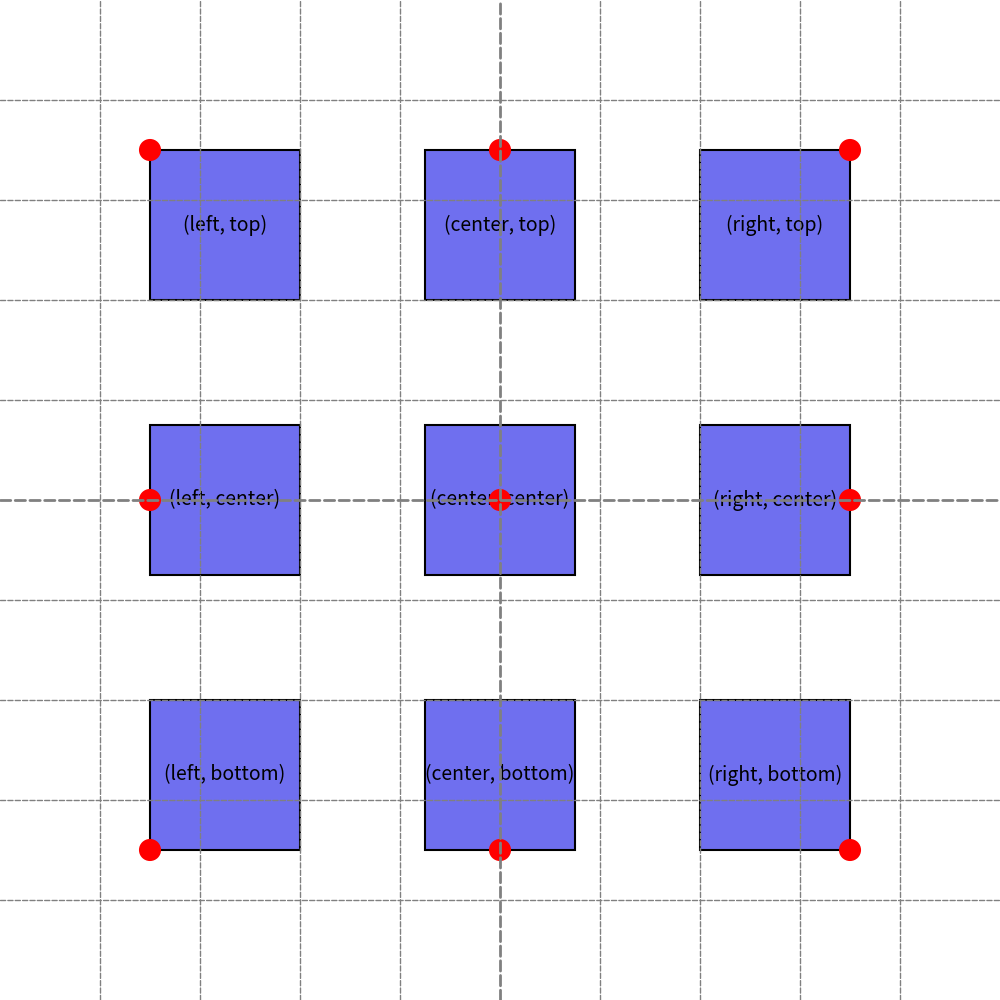
Alignment variations.
We prefer using (center, center)
and (left, bottom)
.
Occasionally, we employ (left, center)
and (center, bottom)
.
(left, bottom)
is beneficial for pinpointing the exact location of rectangle-like shape items.
However, center alignment is much simpler for aligning different-sized multiple items horizontally or vertically.
That’s why we’ve set (center, center)
as the default for all items.
Consistency is key.
Here’s an example of aligning items horizontally and vertically:
1from drawlib.apis import *
2
3config(width=100, height=100, grid_only=True)
4
5x1 = 20
6x2 = 50
7x3 = 80
8y1 = 20
9y2 = 50
10y3 = 80
11
12circle((x1, y1), radius=5)
13rectangle((x1, y2), width=20, height=10, angle=45)
14star((x1, y3), 5, 15, 6, angle=45)
15
16image((x2, y1), width=20, image="python.png", angle=315)
17icon_phosphor.heart((x2, y2), 10, angle=315)
18text((x2, y3), "Drawlib", angle=315, style=TextStyle(size=24))
19
20chevron((x3, y1), 35, 10, corner_angle=45, angle=45)
21parallelogram((x3, y2), 15, 10, corner_angle=60, angle=45)
22regularpolygon((x3, y3), 5, num_vertex=6, angle=45)
23
24for x in [x1, x2, x3]:
25 for y in [y1, y2, y3]:
26 circle((x, y), 1, style=ShapeStyle(fcolor=Colors.Red, lcolor=Colors.Red))
27
28save()
Each item have different size an angles.
If we use alignment like (left, bottom)
, aligning items becomes complex.
However, (center, center)
is straightforward.
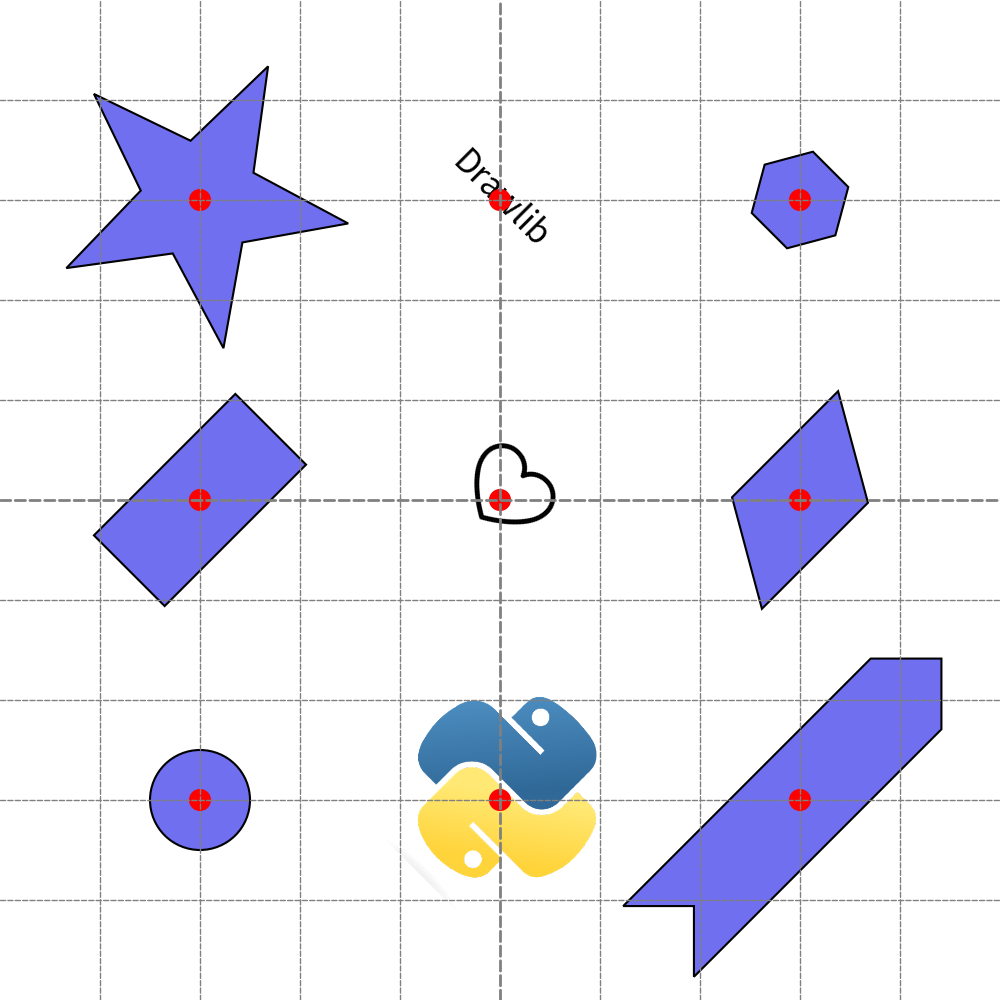
Align center, center is recommended
Please consider the best alignment for placing items. It depends on the situation.