Drawing line
Drawlib provides six functions for drawing lines:
line()
line_curve()
line_bezier1()
line_bezier2()
lines()
lines_bezier()
We will explain each of these functions in detail. They all share the following optional arguments:
arrowhead
: Specifies the type of arrowhead. Options are["", "->", "<-", "<->"]
.width
: Specifies the line width. This should typically be configured within the style, but it is also available as an optional argument.style
: Defines the line style. Accepts a LineStyle object or a string (style name).
Details on these options will be covered in the next section on line styles (see the following page).
line()
The line()
function is the most basic function for drawing lines.
It requires two mandatory arguments and accepts three optional arguments.
xy1: The start point of the line
xy2: The end point of the line
(optional) arrowhead: Specifies the type of arrow head
(optional) width: The width of the line
(optional) style: The style of the line
Let’s look at an example:
1from drawlib.apis import *
2
3config(width=100, height=50)
4line(xy1=(10, 10), xy2=(90, 40))
5save()
In this example, we draw a line from (10, 10) to (90, 40) without specifying a style. This generates the following output:
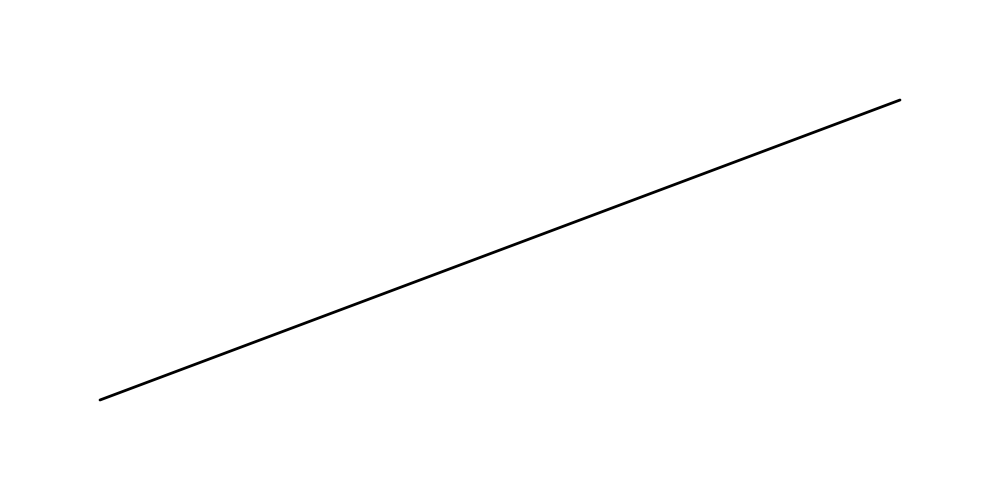
straight line from xy1 to xy2
line_curved()
The line_curved()
function makes it easy to draw curved lines.
While line_bezier1()
and line_bezier2()
can also draw curved lines, they require more complex curve control compared to line_curved()
.
It requires three mandatory arguments and accepts three optional arguments.
xy1: The start point of the line
xy2: The end point of the line
bend: The additional length beyond a direct connection, controlling the curvature.
(optional) arrowhead: Specifies the type of arrow head
(optional) width: The width of the line
(optional) style: The style of the line
For example, suppose xy1 is (10, 10) and xy2 is (10, 20). The distance between these points is 10 units.
When
bend
is set to 0.2, the line is drawn from (10, 10) to (10, 20) with a length of 12 units (10 x 1.2).When
bend
is set to 0.4, the length is 14 units (10 x 1.4).
Negative values can also be used for bend, which maintains the length but reverses the bending direction. Let’s check some examples:
1from drawlib.apis import *
2
3config(width=100, height=50)
4line_curved(xy1=(10, 20), xy2=(90, 20), bend=0.4)
5text((50, 7), "0.4")
6line_curved(xy1=(10, 23), xy2=(90, 23), bend=0.2)
7text((50, 18), "0.2")
8line_curved(xy1=(10, 27), xy2=(90, 27), bend=-0.2)
9text((50, 32), "-0.2")
10line_curved(xy1=(10, 30), xy2=(90, 30), bend=-0.4)
11text((50, 43), "-0.4")
12save()
This code generates the following output:
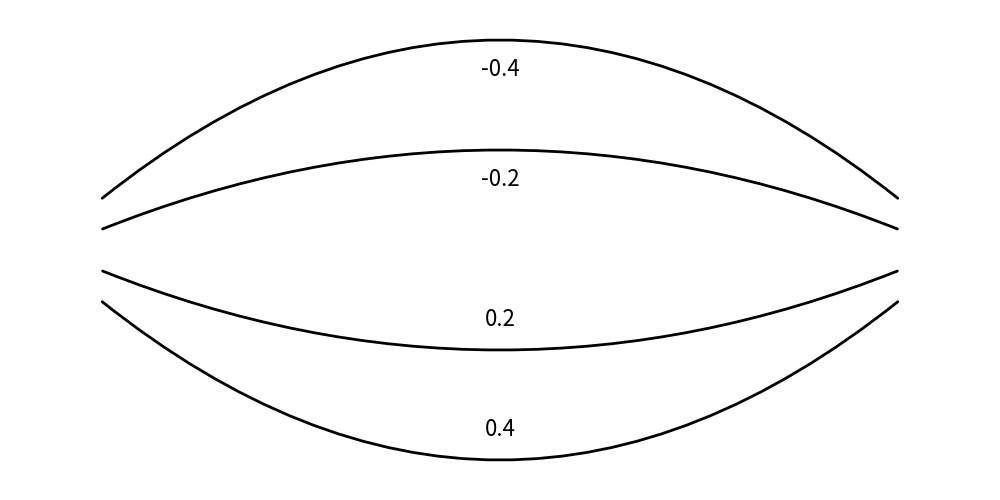
curved line from xy1 to xy2
line_bezier1()
The line_bezier1()
function draws a Bézier curve with one control point.
It requires three mandatory arguments and accepts three optional arguments.
xy1: The start point of the line
cp: The Bézier control point.
xy2: The end point of the line
bend: The additional length beyond a direct connection, controlling the curvature.
(optional) arrowhead: Specifies the type of arrow head
(optional) width: The width of the line
(optional) style: The style of the line
Bézier curves are a popular method for drawing smooth, curved lines. If you are not familiar with Bézier curves, it is recommended to research and understand the concept first.
This code generates the following output:
1from drawlib.apis import *
2
3config(width=100, height=50)
4line_bezier1(xy1=(10, 10), cp=(10, 40), xy2=(40, 40))
5line(xy1=(10, 10), xy2=(10, 40), style=LineStyle(style="dashed", color=Colors.Red))
6line(xy1=(10, 40), xy2=(40, 40), style=LineStyle(style="dashed", color=Colors.Red))
7circle(xy=(10, 10), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
8circle(xy=(10, 40), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
9circle(xy=(40, 40), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
10
11line_bezier1(xy1=(60, 40), cp=(90, 40), xy2=(90, 10))
12line(xy1=(60, 40), xy2=(90, 40), style=LineStyle(style="dashed", color=Colors.Red))
13line(xy1=(90, 40), xy2=(90, 10), style=LineStyle(style="dashed", color=Colors.Red))
14circle(xy=(60, 40), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
15circle(xy=(90, 40), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
16circle(xy=(90, 10), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
17
18save()
It generate this output.
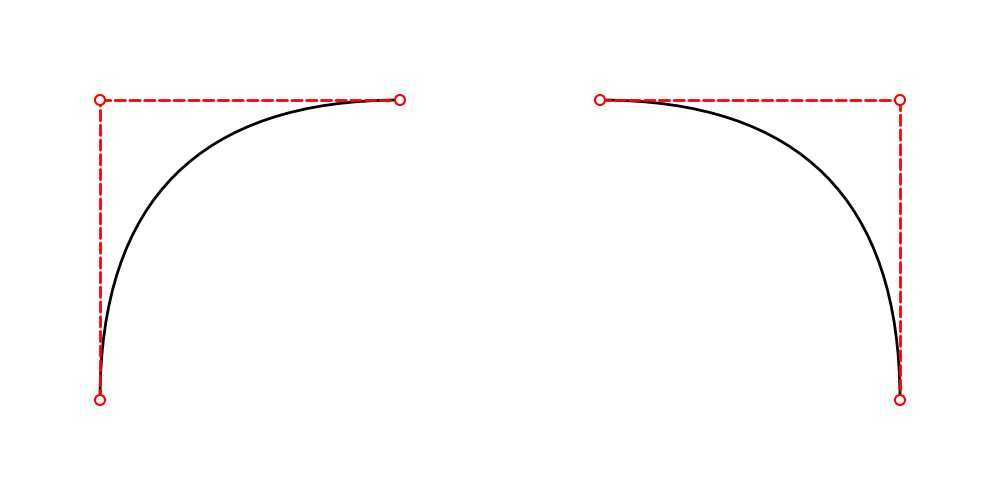
Bézier curves from xy1 to xy2
line_bezier2()
The line_bezier2()
function draws a Bézier curve with two control points.
It requires four mandatory arguments and accepts three optional arguments.
xy1: The start point of the line
cp1: The first Bézier control point.
cp2: The seconde Bézier control point.
xy2: The end point of the line
bend: The additional length beyond a direct connection, controlling the curvature.
(optional) arrowhead: Specifies the type of arrow head
(optional) width: The width of the line
(optional) style: The style of the line
Drawing a Bézier curve with two control points is a popular method for creating smooth, curved lines. If you are not familiar with Bézier curves, it is recommended to research and understand the concept first.
Here is an example code:
1from drawlib.apis import *
2
3config(width=100, height=50)
4line_bezier2(xy1=(10, 10), cp1=(10, 40), cp2=(40, 40), xy2=(40, 10))
5line(xy1=(10, 10), xy2=(10, 40), style=LineStyle(style="dashed", color=Colors.Red))
6line(xy1=(10, 40), xy2=(40, 40), style=LineStyle(style="dashed", color=Colors.Red))
7line(xy1=(40, 40), xy2=(40, 10), style=LineStyle(style="dashed", color=Colors.Red))
8circle(xy=(10, 10), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
9circle(xy=(10, 40), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
10circle(xy=(40, 40), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
11circle(xy=(40, 10), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
12
13line_bezier2(xy1=(60, 40), cp1=(60, 10), cp2=(90, 10), xy2=(90, 40))
14line(xy1=(60, 40), xy2=(60, 10), style=LineStyle(style="dashed", color=Colors.Red))
15line(xy1=(60, 10), xy2=(90, 10), style=LineStyle(style="dashed", color=Colors.Red))
16line(xy1=(90, 10), xy2=(90, 40), style=LineStyle(style="dashed", color=Colors.Red))
17circle(xy=(60, 40), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
18circle(xy=(60, 10), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
19circle(xy=(90, 10), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
20circle(xy=(90, 40), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
21
22save()
Executing this code generates the following output:
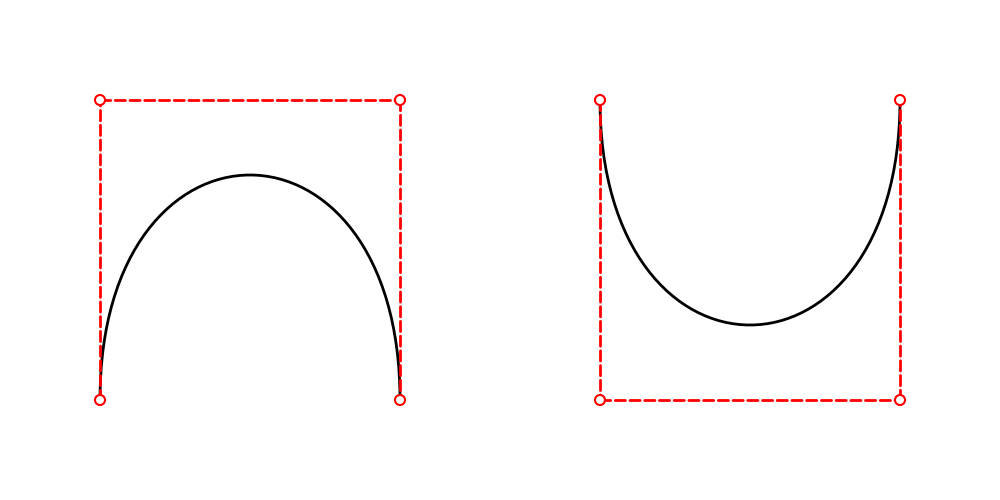
Bézier curves from xy1 to xy2
line_arc()
The line_arc()
function draws a line on the ellipse arc. If the width and height of the ellipse are same, line will be drawn on circle arc.
lines()
The lines()
function draws a line that passes through a series of provided points
It requires one mandatory arguments and accepts three optional arguments.
xys: A list of (x, y) tuples representing the points the line should pass through.
(optional) arrowhead: Specifies the type of arrow head
(optional) width: The width of the line
(optional) style: The style of the line
The xys
argument differs from the previous functions, but it is simply a list of (x, y) coordinates, such as [(10, 10), (20, 40), (30, 10), (40, 40)]
.
Here is an example code:
1from drawlib.apis import *
2
3config(width=100, height=50)
4lines(
5 xys=[
6 (10, 10),
7 (10, 20),
8 (40, 30),
9 (40, 40),
10 (60, 40),
11 (90, 10),
12 ]
13)
14save()
It generate this output.
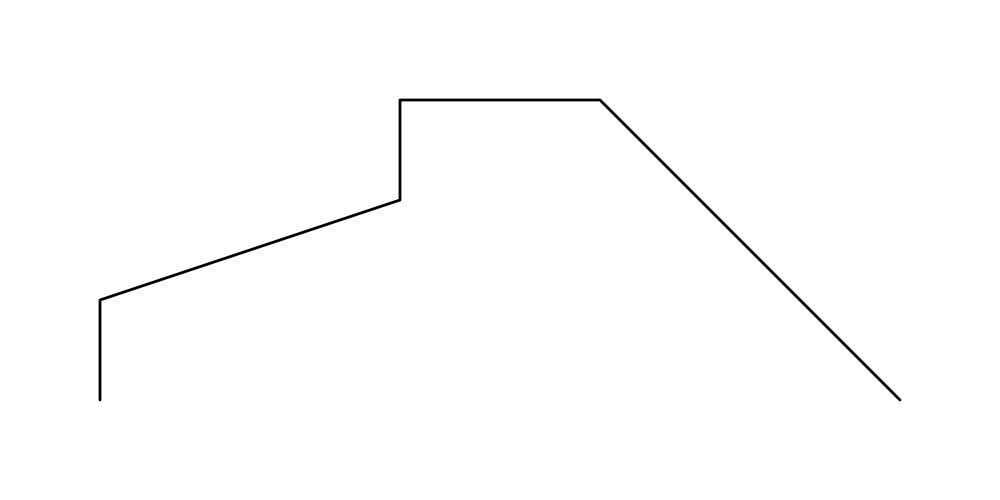
lines which passes list of xy
lines_bezier()
The lines_bezier()
function is similar to lines()
, but it can draw multiple straight lines,
Bézier curves with one control point (bezier1), or Bézier curves with two control points (bezier2) from point to point.
It takes two mandatory arguments and three optional arguments.
xy: The starting point.
path_points: A list of tuples defining the path.
(optional) arrowhead: Specifies the type of arrow head
(optional) width: The width of the line
(optional) style: The style of the line
The path_points
argument can be complex, as it accepts three types of tuples:
(x, y): Draws a straight line from the last point to (x, y).
((cp_x, cp_y), (x, y)): Draws a bezier1 line from the last point to (x, y) with one control point (cp_x, cp_y).
((cp1_x, cp1_y), (cp2_x, cp2_y), (x, y)): Draws a bezier2 line from the last point to (x, y) with two control points (cp1_x, cp1_y) and (cp2_x, cp2_y).
Element of path_points
are very similar to the previous functions line(), line_bezier1(), and line_bezier2().
We set almost the same arguments for elements of the path_points.
Let’s see how it works with an example:
1from drawlib.apis import *
2
3config(width=100, height=50)
4
5points = [
6 ((10, 20), (30, 20)),
7 (60, 10),
8 ((60, 40), (90, 40), (90, 10)),
9]
10lines_bezier(xy=(10, 40), path_points=points)
11
12# bezier1 help line
13line(xy1=(10, 40), xy2=(10, 20), style=LineStyle(style="dashed", color=Colors.Red))
14line(xy1=(10, 20), xy2=(30, 20), style=LineStyle(style="dashed", color=Colors.Red))
15circle(xy=(10, 40), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
16circle(xy=(10, 20), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
17circle(xy=(30, 20), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
18
19# bezier2 help line
20line(xy1=(60, 10), xy2=(60, 40), style=LineStyle(style="dashed", color=Colors.Red))
21line(xy1=(60, 40), xy2=(90, 40), style=LineStyle(style="dashed", color=Colors.Red))
22line(xy1=(90, 40), xy2=(90, 10), style=LineStyle(style="dashed", color=Colors.Red))
23circle(xy=(60, 10), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
24circle(xy=(60, 40), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
25circle(xy=(90, 40), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
26circle(xy=(90, 10), radius=0.5, style=ShapeStyle(fcolor=Colors.White, lcolor=Colors.Red))
27
28save()
In this example, we use all three types of tuples as elements of path_points. They will create a straight line, a bezier1 line, and a bezier2 line.
Executing this code generates the following output:
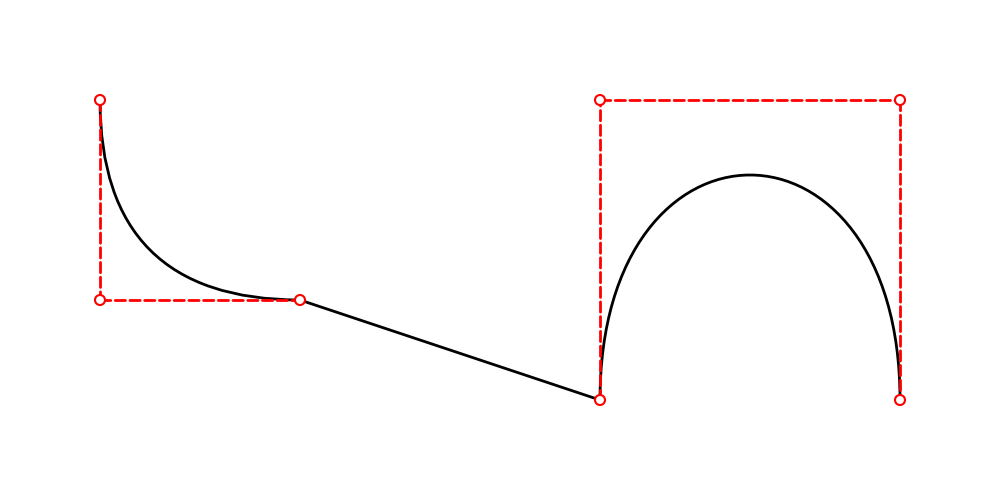
image6.png
This function can be used to draw curved lines from shape to shape like this:
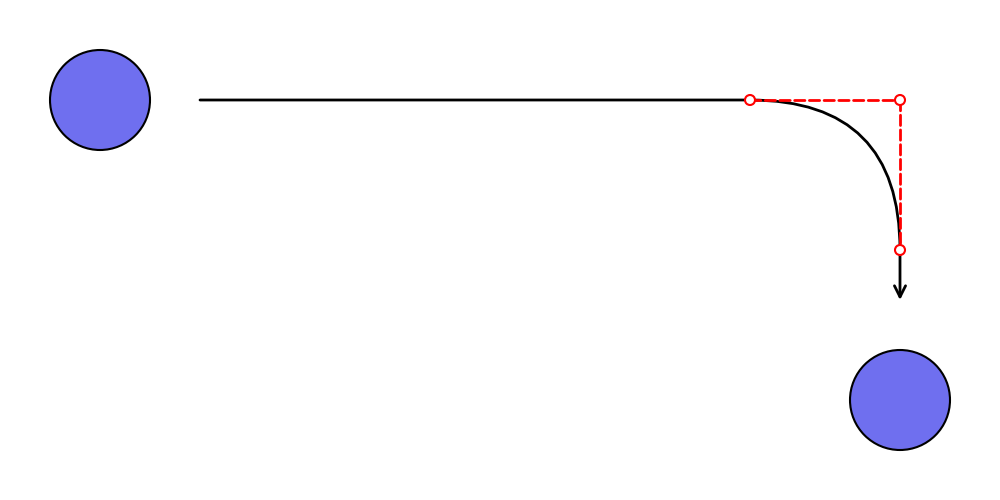
image7.png
For precise control, use this function.
However, if you want to draw a simple curved line, we recommend using lines_curved()
instead.
lines_curved()
The lines_curved()
function is a simplified syntax for lines_bezier()
.
From an argument perspective, this function is almost the same as lines()
, but it includes an additional argument r
which specifies the length of the curve.
This automatically applies a bezier1 curve effect to lines with the specified length r
.
If you want to add related curves to all vertices, this function is very useful.
It takes two mandatory arguments and three optional arguments.
xys: A list of (x, y) tuples representing the points the line should pass through.
r: The length of the curve.
(optional) arrowhead: Specifies the type of arrow head
(optional) width: The width of the line
(optional) style: The style of the line
Here is an example code:
1from drawlib.apis import *
2
3config(width=100, height=50)
4
5circle((10, 40), radius=5)
6lines(
7 [(20, 40), (30, 40), (30, 10), (90, 40), (90, 22)],
8 style=LineStyle(color=Colors.Red, style="dashed", width=1.5),
9)
10lines_curved(
11 [(20, 40), (30, 40), (30, 10), (90, 40), (90, 20)],
12 r=8,
13 width=2.5,
14 arrowhead="->",
15)
16circle((90, 10), radius=5)
17save()
Executing this code generates the following output:
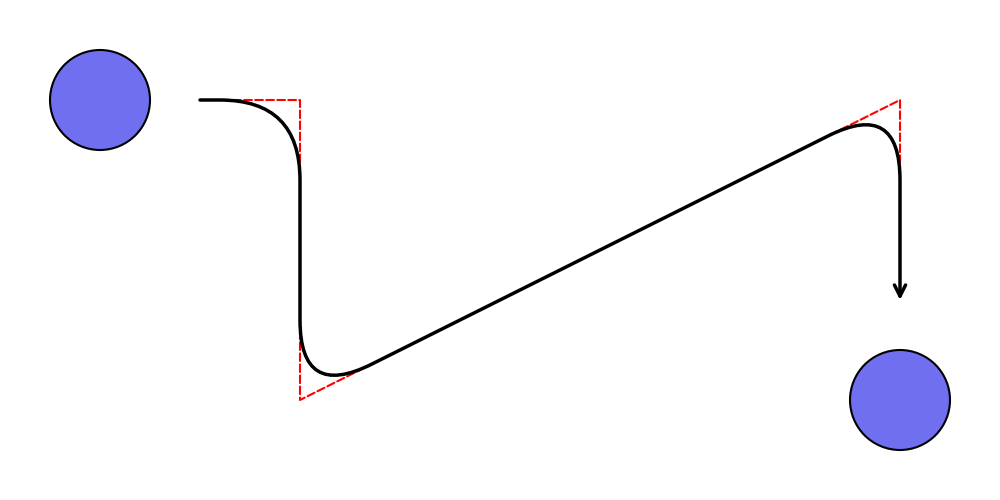
lines_curve()
The red dashed support line length is the value of r
.
If you set a large value, the curve becomes bigger.
However, be careful: r
should be smaller than the distance between points.